NoLang Endpoints
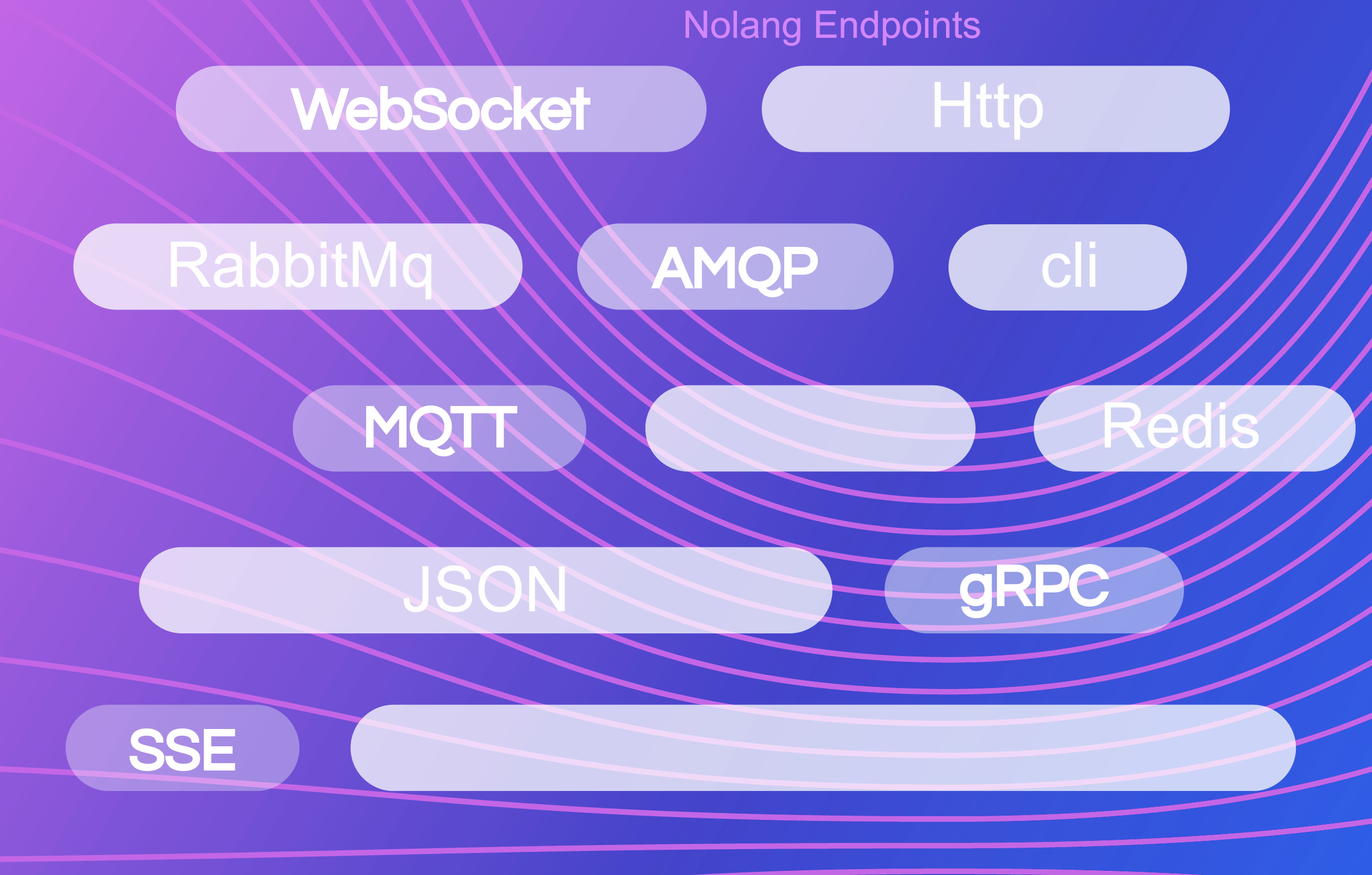
Similar to an API, a NoLang Endpoint is a communication channel that listens to third parties such as webpages, other softwares or even another NoLang applications, for sending commands or queries, use NoLang Scripts.
NoLang supports several types of endpoints:
NoLang supports several types of endpoints:
- list
- http
- socket
- cli
- file
- js
- json
- mqtt
- redis
- rabbitmq
- grpc
- ...
NoLang Http Endpoint (Easy RESTful API)
As a RESTful API, NoLang can be connected via a http endpoint. It is based on the Express Framework. It is possible for an http endpoint to have multiple routing options. Routing refers to how applications respond to client requests for URIs (or paths) and HTTP methods (GET, POST, and so on). For example in below NoLang app setting, there is one endpoint by type http which is listening port 1000 :
{
"endpoints": [
{
"type": "http",
"static": "./public",
"port": 1000,
"cors": {
"origin": "*"
},
"filter": {
"ips": [
"192.200.1.12"
],
"mode": "deny"
},
"routes": [
{
"path": "/",
"method": "post"
},
{
"path": "/tasks/list",
"method": "get",
"type": "html",
"return": {
"$$schema": "task",
"$$header": {
"action": "R"
}
}
},
{
"path": "/history/:key/:value",
"method": "get",
"return": {
"$$schema": "history",
"$$header": {
"action": "R",
"filter": {
"{{env.request.params.key}}": "{{env.request.params.value}}"
},
"user": {
"username": "{{env.request.headers.username}}",
"password": "{{env.request.headers.password}}"
}
}
}
},
{
"path": "/ws",
"method": "ws"
},
{
"path": "/formSubmitTarget",
"method": "post",
"bodyParser": "urlencoded",
"$$header": {
"action": "C"
},
"Field1": "{{env.request.body.Input1}}",
"Field2": "{{env.request.body.Input2}}"
}
]
}
]
}
As you see in above endpoint there is five paths, a POST to receive any NoLang Script, and a path by url /task/list with GET method to return the list of tasks as a result of a pre defined NoLang Script. In the third path we see how to set parameters of request in the script. In the fourth path there is a websocket handler. And in the fifth path we can post a HTML form.
$$res in the response
For sending some options in http response we can use
$$res
in the response of the script. structure of $$res is like bellow:{
"foo": "bar",
"$$res": {
"cookies": {
"cookie1": "value1",
"cookie2": {
"value": "value2",
"options": {}
}
},
"clearCookie": {
"name": {
"path": "/admin"
},
"name2": {}
},
"headers": {
"Set-Cookie": "foo=bar; Path=/; HttpOnly",
"Link": [
" ",
" "
]
},
"attachment": "path/to/logo.png",
"download": "path/to/file.pdf",
"links": {
"next": "http://api.example.com/users?page=2",
"last": "http://api.example.com/users?page=5"
},
"location": "http://example.com",
"type": "jsonp",
"vary": "User-Agent",
"status": 404,
"sendFile": "/absolute/path/to/404.png"
}
}
NoLang Cli Endpoint
With cli endpoints we can send scripts to a NoLang app in the command prompt.
{
"endpoints": [
{
"type": "cli"
}
]
}
If there is a cli endpoint in an app, by running the app in a console, you can send Nolang Scripts for it.
nolang app.json [ENTER]
//type a Nolang script then press ENTER
{"$$schema": "students", "$$header": {"action": "R"}} [ENTER]
//result:
[
{"firstname": "Noora", "lastname": "Salar"} ,
{"firstname": "Fatemeh", "lastname": "Davari"}
]
Scripts can be writen in JSON5 format, and it is possible to see the result in table format if
$$header.view
is equal to 'table'. nolang app.json [ENTER]
//type a Nolang script then press ENTER
{$$schema: students, $$header: {action: R , view: 'table'}} [ENTER]
//result:
--------------------------------
| index | firstname | lastname |
|-------|-----------|----------|
| 0 | Noora | Salar |
| 1 | Fatemeh | Davari |
--------------------------------
NoLang Socket Endpoint
With socket endpoints we can send scripts to a NoLang app through a socket.
{
"endpoints": [
{
"type": "socket",
"port": 1199
}
]
}
NoLang JSON Endpoint
When our app starts, it can run a NoLang script using json endpoints.
{
"endpoints": [
{
"type": "json",
"script": {
"$$schema": "log-schema",
"$$header": {
"action": "C"
},
"event": "starting app",
"time": 1736611339373
}
}
]
}
NoLang File Endpoint
Similar to json endpoints, when our app starts, it can run a NoLang script in a .json or .json5 file using file endpoints.
{
"endpoints": [
{
"type": "file",
"filename": "../scripts/startup-script.json5"
}
]
}
NoLang Js Endpoint
Similar to file endpoints, when our app starts, it can run a NoLang script exported from a .js file using js endpoints.
{
"endpoints": [
{
"type": "js",
"filename": "../scripts/startup-script.js"
}
]
}
The startup-script.js file can be like below:
module.exports = async function (endpoint){
let res1 = await endpoint({
$$schema: 'students',
$$header: {
action: 'R',
filter: {
firstname: 'Fatemeh'
}
}
})
console.log(res1[0].firstname)
}
NoLang Redis Endpoint
A NoLang app can be connected with redis endpoints, It is very useful for microservices.
{
"endpoints": [
{
"type": "redis",
"url": "redis://localhost:6380",
"subscribeChannels": "App1*response",
"publishChannels": "App1*request"
}
]
}
According to the sample above, the Nolang app has a redis endpoint containing two channels,
Subscribing to
subscribeChannels
allows the app to receive scripts from clients, and publish responses to channels named in publishChannels
. We can see more information in microservices later.NoLang MQTT Endpoint
A NoLang app can be connected with mqtt endpoints, It is also very useful for microservices.
{
"endpoints": [
{
"type": "mqtt",
"url": "mqtt://test.mosquitto.org",
"subscribeTopic": "chatroom/+/res",
"publishTopic": "chatroom/+/req"
}
]
}
According to the sample above, the app has a mqtt endpoint with two topics,
Subscribing to
subscribeTopic
allows the app to receive scripts from clients, and publishing responses for the channels named in publishTopic
. We can see more information in microservices later.